As a developer, you might have encountered concurrency issues such as race conditions and deadlocks in your C# code. These issues can be challenging to debug and can lead to unpredictable behavior in your program. Race conditions occur when multiple threads access the same shared resource, leading to unexpected behavior due to a lack of synchronization between the threads. Deadlocks happen when two or more threads are waiting for each other to release a resource, causing a deadlock in the program.
In this blog, we will discuss the common pitfalls of race conditions and deadlocks in C# and how to avoid them. We will cover the best practices that you can use to write robust and efficient multi-threaded code. So, let’s dive in and explore the world of concurrency in C#.
Avoiding Race Conditions in C# Programs
Race conditions can cause unexpected and erroneous behavior in programs because the result of an operation depends on which thread executes it first. In C#, race conditions can occur in any situation where multiple threads access shared resources, such as global variables, objects, or files.
The most common way to avoid race conditions is to use synchronization techniques, such as locks or semaphores, that ensure only one thread can access a shared resource at a time. Additionally, C# provides built-in language features such as the ‘volatile’ keyword, which can be used to ensure that the latest value of a variable is always read by a thread, preventing race conditions from occurring.
To avoid race conditions in C#, you can use synchronization techniques to ensure that only one thread can access a shared resource at a time. Here are some strategies to consider:
Locks:
One common synchronization technique is to use locks to restrict access to a shared resource. An object that can only be held by one thread at a time is a lock. A thread must obtain the lock before accessing a shared resource, and it must release the lock once it is finished. By doing this, race conditions are avoided, and it is ensured that only one thread can access the resource at once.
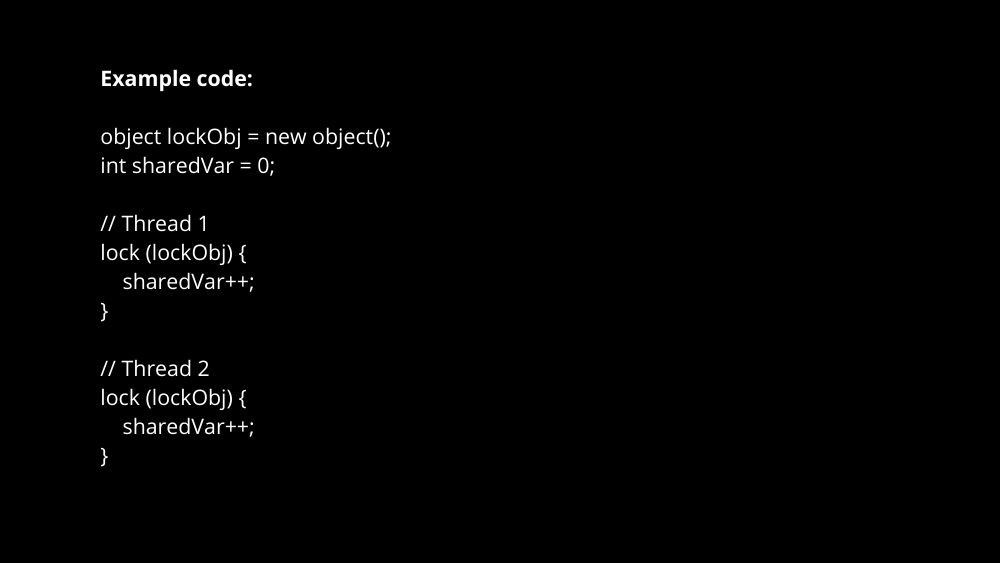
In this example, two threads access the shared variable ‘sharedVar’ by acquiring a lock on the object ‘lockObj’. By using locks, only one thread can access ‘sharedVar’ at a time, avoiding race conditions.
Interlocked:
Another way to avoid race conditions is to use the ‘Interlocked’ class, which provides atomic operations on shared variables. Atomic operations are those that are performed in a single step, without interruption from other threads. This ensures that the operation is completed before any other thread can access the shared variable, preventing race conditions.
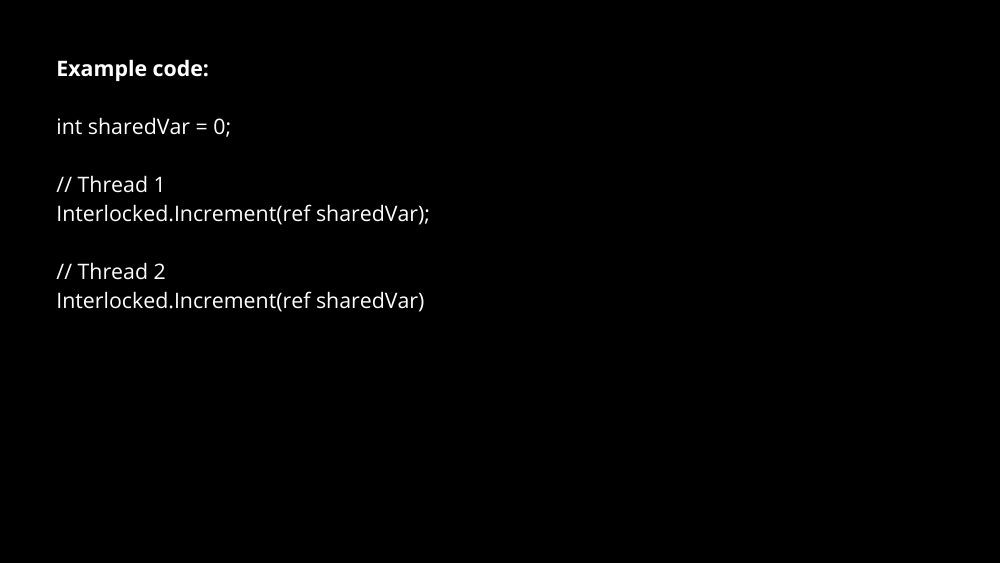
In this example, two threads increment the shared variable ‘sharedVar’ using the ‘Interlocked.Increment’ method. This method ensures that the increment operation is performed atomically, avoiding race conditions.
Thread Safety:
When writing C# code, it’s important to consider thread safety when designing classes and methods. Thread-safe code is code that can be safely accessed by multiple threads simultaneously without causing race conditions. You can ensure thread safety by using synchronization techniques, such as locks or Interlocked operations, or by using immutable objects that cannot be modified once they are created.
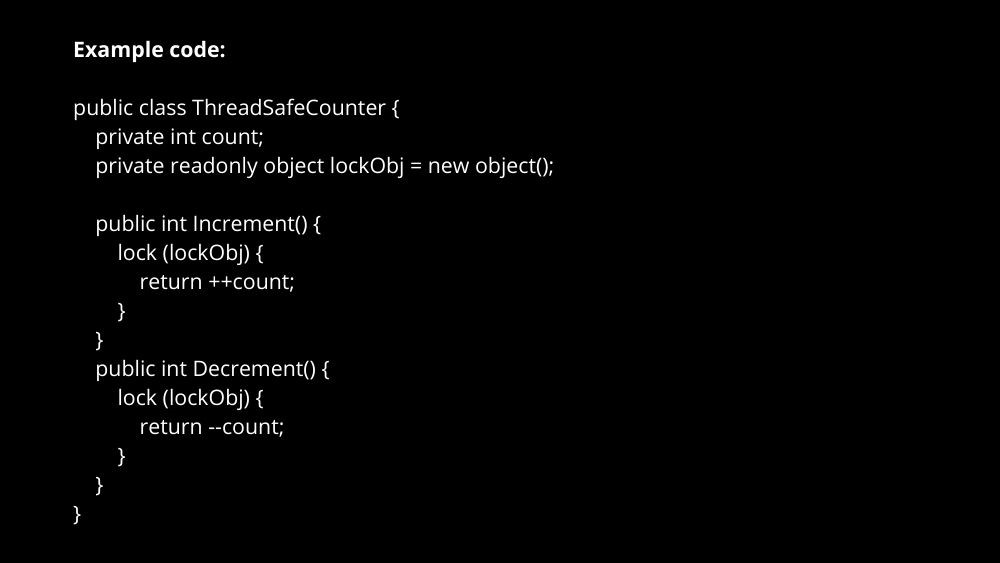
In this example, a thread-safe counter class is implemented using locks to ensure that the ‘Increment’ and ‘Decrement’ methods can be safely accessed by multiple threads without causing race conditions.
In conclusion, avoiding race conditions in C# requires careful consideration of how shared resources are accessed by multiple threads. By using synchronization techniques, such as locks or Interlocked operations, or by designing thread-safe classes and methods, you can avoid race conditions and ensure that your C# code behaves predictably and correctly in a multi-threaded environment.
Avoiding Deadlocks in C# Programs
Deadlock is a situation in concurrent computing where two or more threads or processes are blocked, waiting for each other to release resources that they need to proceed, resulting in a circular wait. Essentially, each process is waiting for the other process to release a resource before it can continue. As a result, both processes end up waiting indefinitely, effectively halting the entire system. It can be difficult to detect and can lead to severe performance degradation or even system crashes. Therefore, it’s essential to identify and prevent deadlocks in concurrent systems.
Deadlocks can be prevented in C# programs by following some best practices and using synchronization techniques. Here are some strategies to consider:
Avoid Circular Dependencies:
Circular dependencies occur when two or more threads or processes are waiting for resources held by each other. This situation can be avoided by designing the system such that each process acquires resources in a specific order and releases them in the reverse order. This ensures that there is no circular wait and that deadlock can be prevented.
Use Timeouts:
When acquiring resources, use timeouts to avoid waiting indefinitely. If a resource is not available within a certain time frame, the thread should release all acquired resources and try again later.
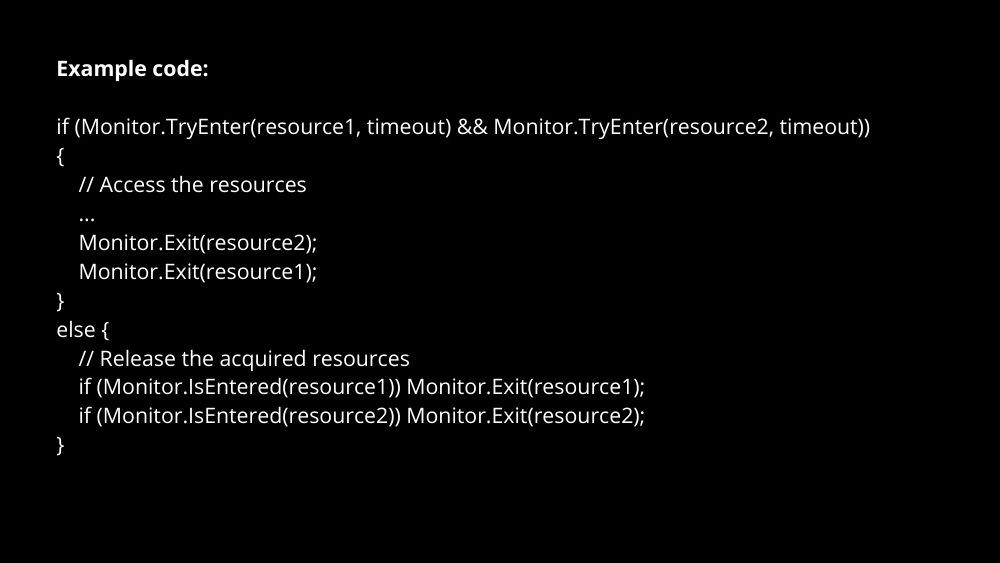
In this example, the ‘Monitor.TryEnter’ method is used to acquire the resources ‘resource1’ and ‘resource2’ with a timeout. If the resources are not available within the timeout period, the thread releases all acquired resources and tries again later.
Use Lock Hierarchy:
Use a lock hierarchy to prevent circular dependencies. A lock hierarchy is a set of locks arranged in a specific order, and each thread acquires locks in the same order. This ensures that there is no circular wait and that deadlock can be prevented.
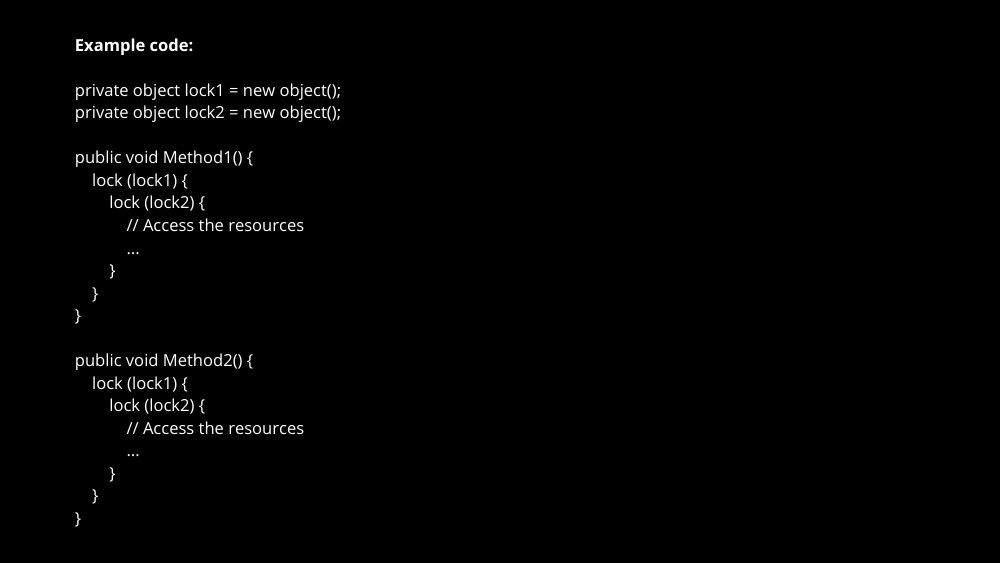
In this example, the methods ‘Method1’ and ‘Method2’ acquire the locks ‘lock1’ and ‘lock2’ in the same order, ensuring that there is no circular wait and that deadlock can be prevented.
Use the Task Parallel Library (TPL):
The Task Parallel Library (TPL) is a powerful concurrency framework provided by .NET that can help prevent deadlocks. TPL uses tasks to represent units of work, and tasks can be composed to create complex workflows. TPL automatically manages concurrency and ensures that tasks do not interfere with each other, preventing deadlocks.
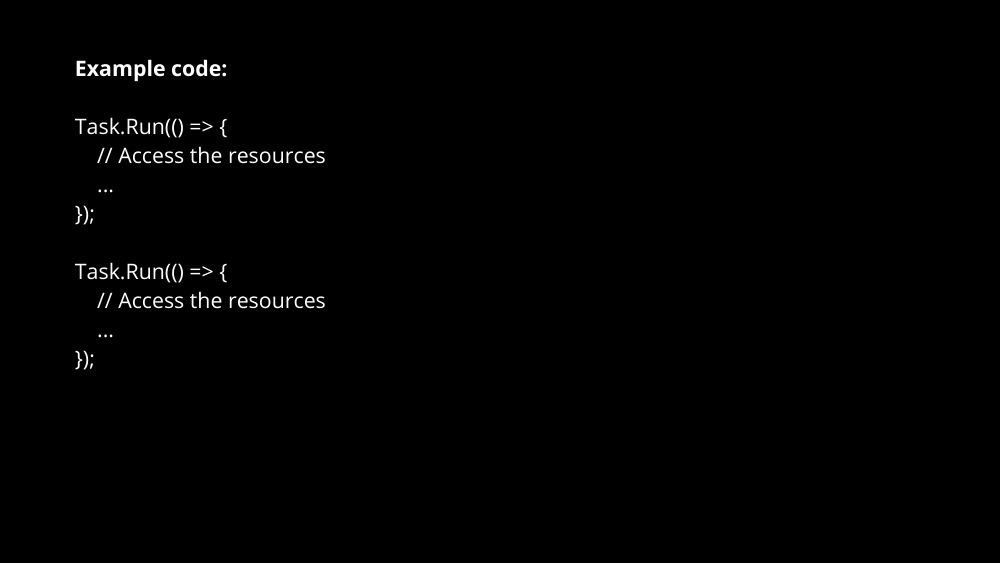
In this example, two tasks are created using the ‘Task.Run’ method, which automatically manages concurrency and ensures that the tasks do not interfere with each other, preventing deadlocks.
In conclusion, preventing deadlocks in C# requires careful consideration of how resources are acquired and released in a multi-threaded environment. By following best practices and using synchronization techniques, such as timeouts, lock hierarchy, and the Task Parallel Library, you can prevent deadlocks and ensure that your C# code behaves predictably and correctly in a concurrent system.